|
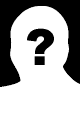 Tom Schaefer | 2013-05-25 19:54:09 |
Your last commit is really neat. Drive it further and you have written a jquery excel app.
2ThumbsUp!! |
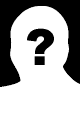 ikhsan | 2013-05-25 22:32:22 - In reply to message 1 from Tom Schaefer |
Hi Tom,
Thanks, It's still far away to be called 'excel app' :)
just let me know if you found any bug, or kindly fork it on github if you are interested
https://github.com/ikhsan017/calx |
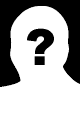 Tom Schaefer | 2013-05-26 08:14:03 - In reply to message 2 from ikhsan |
Yes, I know that this script is rudimentary. But you are using a grammar like style. This is a new way. All the other jquery bound excel-imitating frameworks are working another way. The more the grammar process is solving, the less you have to implement. Over-think what I am writing! |
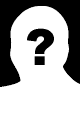 Tom Schaefer | 2013-05-26 08:49:39 - In reply to message 1 from Tom Schaefer |
median: function (a, b) {
var $val = [];
var $start = ('' + a).split('.');
var $stop = ('' + b).split('.');
for (a in $start) {
$start[a] = parseInt($start[a]);
$stop[a] = parseInt($stop[a]);
}
var $c_start = ($start[0] < $stop[0]) ? $start[0] : $stop[0];
var $c_stop = ($start[0] > $stop[0]) ? $start[0] : $stop[0];
var $r_start = ($start[1] < $stop[1]) ? $start[1] : $stop[1];
var $r_stop = ($start[1] > $stop[1]) ? $start[1] : $stop[1];
for (col = $c_start; col <= $c_stop; col++) {
for (row = $r_start; row <= $r_stop; row++) {
var $rowIndex = utility.toChr(col) + row;
var $cellValue = calx.matrix[formula.key].value[$rowIndex];
$cellValue = ($cellValue) ? parseFloat($cellValue) : 0;
$val.push($cellValue);
}
}
var median = 0,
numsLen = $val.length;
$val.sort();
if (numsLen % 2 === 0) { // is even
// average of two middle numbers
median = ($val[numsLen / 2 - 1] + $val[numsLen / 2]) / 2;
} else { // is odd
// middle number only
median = $val[(numsLen - 1) / 2];
}
return median;
},
|
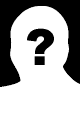 Tom Schaefer | 2013-05-26 08:51:53 - In reply to message 4 from Tom Schaefer |
I suggest to implement a array helper object which reduces duplicate code.
@see min,max,media have all following duplicate code :
<code>
var $val = [];
var $start = ('' + a).split('.');
var $stop = ('' + b).split('.');
for (a in $start) {
$start[a] = parseInt($start[a]);
$stop[a] = parseInt($stop[a]);
}
var $c_start = ($start[0] < $stop[0]) ? $start[0] : $stop[0];
var $c_stop = ($start[0] > $stop[0]) ? $start[0] : $stop[0];
var $r_start = ($start[1] < $stop[1]) ? $start[1] : $stop[1];
var $r_stop = ($start[1] > $stop[1]) ? $start[1] : $stop[1];
for (col = $c_start; col <= $c_stop; col++) {
for (row = $r_start; row <= $r_stop; row++) {
var $rowIndex = utility.toChr(col) + row;
var $cellValue = calx.matrix[formula.key].value[$rowIndex];
$cellValue = ($cellValue) ? parseFloat($cellValue) : 0;
$val.push($cellValue);
}
}
</code> |
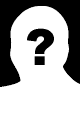 Tom Schaefer | 2013-05-26 08:57:38 - In reply to message 5 from Tom Schaefer |
In only 5 min. I implemented the median function!
Your works is great. You 'd done the basics. So now a little refactoring has to be done to reduce code duplicates.
Means: Implementing of new functionalities is very easy.
I suggest an addon feature for being able to implement futher functionalities in grouped or cluster way:
- statistical (A,B,C)
- financial (A,B,C)
and so on |
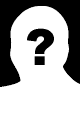 Tom Schaefer | 2013-05-26 09:46:01 - In reply to message 6 from Tom Schaefer |
<code>
var arrayHelper = {
computeValues: function(a, b, mode){
var $val, $count;
if(mode){
$val = 0;
$count = 0;
} else {
$val = [];
}
var $start = ('' + a).split('.');
var $stop = ('' + b).split('.');
for (a in $start) {
$start[a] = parseInt($start[a]);
$stop[a] = parseInt($stop[a]);
}
var $c_start = ($start[0] < $stop[0]) ? $start[0] : $stop[0];
var $c_stop = ($start[0] > $stop[0]) ? $start[0] : $stop[0];
var $r_start = ($start[1] < $stop[1]) ? $start[1] : $stop[1];
var $r_stop = ($start[1] > $stop[1]) ? $start[1] : $stop[1];
for (col = $c_start; col <= $c_stop; col++) {
for (row = $r_start; row <= $r_stop; row++) {
var $rowIndex = utility.toChr(col) + row;
var $cellValue = calx.matrix[formula.key].value[$rowIndex];
if(mode) {
$val += ($cellValue) ? parseFloat($cellValue) : 0;
if(mode==2){
$count++;
}
} else {
$cellValue = ($cellValue) ? parseFloat($cellValue) : 0;
$val.push($cellValue);
}
}
}
return {
val: $val,
count: $count
};
}
}
</code>
<code id="median">
median: function (a, b) {
var $c = arrayHelper.computeValues(a,b);
function median(ary) {
if (ary.length == 0)
return null;
ary.sort(function (a,b){return a - b})
var mid = Math.floor(ary.length / 2);
if ((ary.length % 2) == 1) // length is odd
return ary[mid];
else
return (ary[mid - 1] + ary[mid]) / 2;
}
return median($c.val);
},
</code> |
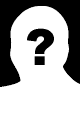 ikhsan | 2013-05-26 09:47:49 - In reply to message 5 from Tom Schaefer |
Yep, I see those duplication and need some refactor
will implement new utility for iterating range cell soon
Great to hear you add more function, I will also create a builder to make modification in parser and formula lot easier |
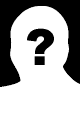 Tom Schaefer | 2013-05-26 09:57:42 - In reply to message 8 from ikhsan |
Good work!
I implemented median, mode and mean in a few minutes after generating the parser code.
|
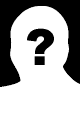 ikhsan | 2013-05-28 00:29:55 - In reply to message 9 from Tom Schaefer |
Hi Tom,
the code you mentioned before, are now refactored to utility.iteraceCell()
formula code should be leaner now, will group the formula later |
|